Writing efficient unit tests in Java: best practices and examples

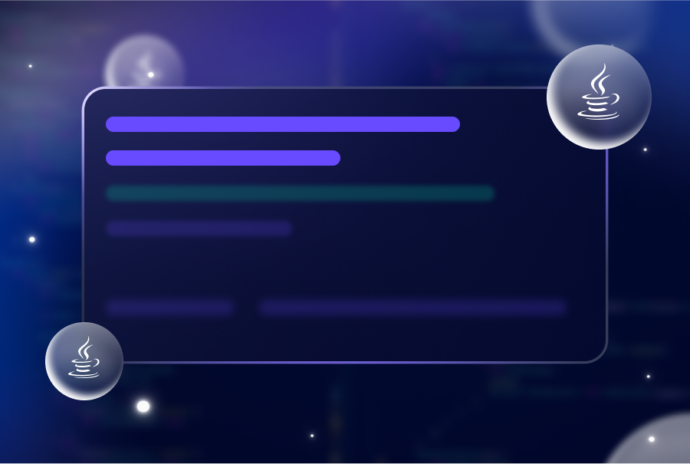
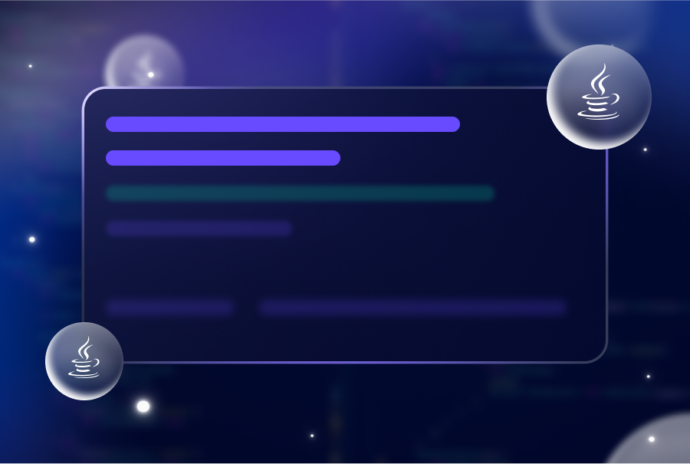
Unit testing is like the essential vegetable on a developer’s plate—crucial for a healthy codebase, yet often pushed aside. However, robust Java applications consistently share one common trait: comprehensive test suites that catch bugs before they ever reach production. These tests provide the first, nearly instant feedback loop for developers, allowing them to confirm if their code functions as expected. While unit tests aren’t a substitute for best practices like the SOLID principles, untestable code is a clear indication that these principles are likely being overlooked..
In modern Java development, unit testing transcends basic code verification — it shapes better software architecture. Intuitively, test-first approaches lead to more modular, loosely coupled code. But making the tests effective requires a deep understanding of testing principles, patterns, and common pitfalls that can make or break a test suite.
This guide cuts through theoretical concepts to deliver practical, actionable techniques for writing efficient unit tests in Java. From improving test coverage to optimizing execution speed and enhancing maintainability, you’ll find concrete examples and field-tested practices to strengthen your testing approach. So, without further ado, let’s dive in.
What are unit tests?
Unit tests are, for most intents and purposes, quality control inspectors on a production line, examining each component before it joins the assembly. In software development, these “components” are the smallest testable pieces of code — typically individual methods or functions that perform specific tasks.
Here’s how different types of tests compare in the testing hierarchy:
Test Type | Scope | Speed | Dependencies |
Unit Tests | Single component | Milliseconds | Isolated/Mocked |
Integration Tests | Multiple components | Seconds | Partial |
E2E Tests | Entire application | Minutes | Complete |
A proper unit test should have these key characteristics:
- Tests run in isolation without external dependencies
- Executes quickly (milliseconds)
- Produces consistent results across multiple runs
- Tests a single piece of functionality
- Order of test execution doesn’t matter
Why do efficient unit tests matter?
Unit testing directly impacts development velocity and code quality in measurable ways. Microsoft’s empirical study of a 32-developer team demonstrated a 20.9% decrease in test defects after implementing automated unit testing practices. While this required approximately 30% more development time initially, the investment proved valuable through reduced customer-reported defects in the following two years.
Development velocity
When developers can verify changes in milliseconds rather than waiting for full system tests, iteration speed increases, creating a rapid feedback loop that then prevents bugs from propagating through the codebase.
Long-term codebase health
These tests also serve as living documentation of code behavior and design decisions, enforcing modularity and loose coupling, as testable code inherently requires clear interfaces and minimal dependencies.
Cost reduction
Early bug detection through unit testing significantly reduces debugging time and prevents costly fixes in production environments.
Setting up a unit testing environment in Java
Let’s examine the key components of a robust unit testing environment and their configurations.
Selecting frameworks
Here’s how popular testing frameworks compare:
Feature | JUnit 5 | TestNG | Spock |
Primary use | Unit testing | Unit & Integration | Unit testing |
Learning curve | Moderate | Steep | Moderate DSL |
Parallel execution | Built-in | Built-in | JUnit Jupiter |
Mocking support | External | External | Built-in – External |
Notably, JUnit 5 stands out for unit testing given its modern architecture and extensive IDE support.
Dependency management
For Maven projects, add this minimal configuration to your pom.xml:
... org.junit.jupiter junit-jupiter 5.9.2 test
For Gradle projects, add to build.gradle:
dependencies { // Main dependencies ... // Testing dependencies testImplementation 'org.junit.jupiter:junit-jupiter:5.9.2' testRuntimeOnly 'org.junit.platform:junit-platform-launcher' } test { useJUnitPlatform() // Enable JUnit 5 testing }
It also comes highly recommended that we use a single version across multiple dependencies, such as here:
// Gradle ext { junitVersion = '5.9.2' } dependencies { testImplementation "org.junit.jupiter:junit-jupiter-api:${junitVersion}" testRuntimeOnly "org.junit.jupiter:junit-jupiter-engine:${junitVersion}" }
This ensures your project has the necessary dependencies while remaining maintainable and clear.
Environment configurations
A well-structured test environment is essential for maintainable and reliable unit tests. Here’s how to set up your testing environment effectively:
- The standard Maven/Gradle project structure separates test code from production code. To wit, place test classes in the src/test/java directory.
- Your test directory should mirror your production code structure. For example:
- Production: src/main/java/com/company/app/service/UserService.java
- Test: src/test/java/com/company/app/service/UserServiceTest.java
- Configure test resources separately in src/test/resources. The resources will cover everything from test configuration files to data files, mock response files, data files, and also test-specific properties.
- Set up logging to capture test output effectively:
// In your test class or base test class @BeforeAll static void setupLogging() { System.setProperty("org.slf4j.simpleLogger.defaultLogLevel", "DEBUG"); }
Principles of writing efficient unit tests
Writing efficient unit tests requires more than just knowing the syntax — it demands a structured approach to ensure tests remain valuable throughout the development lifecycle.
Isolation is key
Unit tests validate behavior in isolation, which means they don’t rely on databases, file systems, or network calls. Instead, they use mocks or stubs to simulate these external dependencies. For example, when testing a user authentication method, you’d mock the database calls rather than calling an actual database.
This isolation serves two critical purposes: it keeps tests fast and reliable, and it ensures that when a test fails, you know exactly which piece of code is responsible. Through dependency injection, tests gain complete control over external dependencies, allowing precise simulation of various scenarios. Plus, mocking frameworks complement this by enabling tests to verify behavior without relying on actual implementations.
When each test manages its own setup and teardown, it becomes truly independent, eliminating the risk of interference between test cases.
Determinism
Deterministic tests are the hallmark of a trustworthy test suite since they produce consistent results regardless of when or where they run. This means replacing time-dependent operations with controlled values and using fixed seeds for any random operations. External factors that could affect test outcomes must be carefully controlled, so that when tests behave predictably, developers can trust their results and quickly identify real issues rather than chasing environmental problems or timing-related failures.
Clarity over cleverness
Complex test logic often obscures the actual behavior being verified, making the tests brittle, maintenance difficult and reducing the test’s value as documentation. Instead, focus on writing straightforward tests that clearly demonstrate intent and at the same time, use descriptive names that explain both the scenario and the expected outcome. Following consistent patterns helps other developers understand and maintain the tests, making the entire codebase more maintainable.
FAST Principles
The FAST principles form the cornerstone of effective unit testing, each element contributing to a robust testing strategy.
- Fast: Tests must execute in milliseconds. This means optimizing execution time, avoiding file I/O operations and network calls, and keeping database operations to a minimum. Fast tests encourage developers to run them frequently, catching issues early in the development cycle.
- Automatic: Tests should run without any manual intervention. This includes automatic test discovery, data setup, and cleanup. Tests should be configured to run on code commits and integrate seamlessly with CI/CD pipelines, providing immediate feedback on code changes.
- Self-verifying: Results must be unambiguous with clear pass/fail outcomes. Tests should use precise assertions that validate specific behaviors and provide meaningful error messages. The intention is to make manual result interpretation moot and redundant.
- Timely: Write tests alongside or before production code to guide development. This practice ensures testability is built into the design from the start and helps maintain high code quality. Regular updates to tests when requirements change keep the test suite relevant and valuable.
Best practices for writing maintainable tests
Keeping in mind the principles for writing efficient unit tests listed above, here are a few practical best practices:
Single responsibility in tests
Each test should verify one specific behavior or scenario. Multiple assertions within a test are acceptable as long as they validate the same logical outcome.
Descriptive naming conventions
Test names should follow a clear pattern that describes the scenario, action, and expected outcome. Here’s a practical example:
@Test void validateEmail_withMalformedAddress_throwsValidationException() { EmailValidator validator = new EmailValidator(); assertThrows(ValidationException.class, () -> validator.validate("invalid.email@")); }
Avoid hard-coded values
Maintain test data in constants or configuration files:
class UserServiceTest { private static final String VALID_EMAIL = "[email protected]"; private static final String VALID_PASSWORD = "Pass123!"; @Test void createUser_withValidCredentials_returnsCreatedUser() { UserService service = new UserService(mockRepository); User user = service.createUser(VALID_EMAIL, VALID_PASSWORD); assertNotNull(user.getId()); assertEquals(VALID_EMAIL, user.getEmail()); } }
Documentation and comments
Document complex business rules or edge cases. For example:
@Test void calculateDiscount_withTieredPricing_appliesCorrectRate() { // Business Rule: Orders > $1000 get 10% discount // Orders > $5000 get additional 5% PriceCalculator calculator = new PriceCalculator(); double price = calculator.calculate(6000.0); assertEquals(5100.0, price); // 6000 - 15% discount }
Avoiding common pitfalls in unit testing
Unit testing can be tricky, and even experienced developers often fall into common traps. Here’s how to avoid them:
Over-mocking
Creating excessive mocks can lead to brittle tests that break with minor code changes. Instead of mocking every dependency, focus on mocking only the essential external dependencies. For instance, when testing a service layer, mock the database calls but use real implementations for utility classes.
Test interdependencies
Tests should never depend on each other’s state or execution order. A separate test environment for each test (that gets cleaned up later automatically) creates an encapsulation that ensures that tests can run in parallel and failures are easier to diagnose. Say, for example, if you’re testing a user registration system, each test should create its own user data rather than relying on data created by other tests.
Too many assertions
As mentioned above, while multiple assertions in a test are acceptable, they should all verify related aspects of the same behavior. If you find yourself testing multiple unrelated conditions, it’s a sign that your test (or possibly the code under test) is doing too much. Keep assertions focused on a single logical outcome.
Ignoring edge cases
Edge cases often reveal critical bugs, yet they’re frequently overlooked. Test boundary conditions, null values, empty collections, and error scenarios. When testing a sorting algorithm, for example, verify behavior with empty lists, single-element lists, and lists with duplicate values.
Tools and techniques to speed up testing
The difference between a test suite that developers love to run and one they avoid often comes down to execution speed and maintenance overhead. Let’s explore some modern tools and techniques that can transform your testing workflow from a time sink into a productive feedback loop.
AI coding tools – Qodo
Qodo brings artificial intelligence to unit testing, fundamentally changing how developers approach test creation. By analyzing code patterns and business logic, it automatically suggests comprehensive test cases that even experienced developers might overlook. But most importantly, Qodo’s strength lies in its ability to identify edge cases and generate tests for complex scenarios given its above-par contextual understanding of code, particularly in Java applications,
Rather than generating generic test templates, it analyzes the actual implementation and suggests tests that validate business-critical paths. Plus, the tool integrates seamlessly with popular IDEs, providing real-time suggestions while developers write the code. The immediate impact, obviously, is the quick feedback loop that helps maintain high test coverage while also reducing the time spent on test creation.
Code coverage tools
JaCoCo provides detailed coverage metrics through its integration with Maven and Gradle, generating HTML reports showing line, branch, and complexity coverage. SonarQube complements JaCoCo by adding quality gates and continuous inspection of code coverage. However, coverage alone is not the complete feedback loop—it is essential to validate that tests meet the intended requirements for each unit of work.
Achieving lower coverage yet ensuring requirements are met could indicate a disconnect; it may point to over-engineered or unnecessary logic that doesn’t directly serve the core requirements. This gap signals potential areas for code simplification, ensuring that tests are directly aligned with business logic rather than covering extraneous functionality.
In this way, a balanced approach is required, focusing on both coverage metrics and alignment with intended requirements.
Test reporting for continuous feedback
Modern CI/CD pipelines can transform raw test results into actionable insights. Tools like Jenkins and GitHub Actions can be configured to display test results prominently, making failures immediately visible to the team.
Parallel testing
For large test suites, parallel execution can dramatically reduce build times. Modern testing frameworks support concurrent test execution, with proper isolation ensuring reliable results.
Conclusion
As we have seen above, unit testing is more art than science — it requires balancing thoroughness with practicality. By following the practices outlined in this guide, from writing focused tests to leveraging modern tools like Qodo, you can build a robust testing strategy that catches bugs early and maintains code quality. At the same time, it’s worth exploring property-based testing for more comprehensive test coverage, or diving deeper into CI/CD integration to automate your testing workflow. Remember: good tests don’t just verify code; they document intent and enable confident refactoring.